Day 9 - July 8, 2022
Topics Covered
- Geolocation
- Requesting user location
- Displaying maps
- Working with maps
Requesting user location
The geolocation API that is used by web applications to figure out where the user is located can also be used by React Native applications. This API is useful for getting the precise coordinates from the GPS on mobile devices. You can use this information for customizing user content or for "big data" analytics.
In the code example, we obtain and display the latitude and longitude from the geolocation API. Normally, displaying this information isn't too helpful for the users of your application. Rather than displaying the coordinates, we could use this information to perform lookups. Maybe we can display information about restaurants or hotels that are nearby.
The getCurrentPosition()
and watchPosition()
functions run asyncronously.
Remember, it is a good practice use the useEffect
hook to prevent unexpected behaviour.
Displaying maps
By importing the react-native-maps
package, we gain access to the MapView
component. MapView
allows us to easily render maps. In this code example, a map containing
a marker at your current location is rendered. In order for the example to work, you may need to grant
location access to your application.
By enabling showsUserLocation
a marker is placed on the map. To keep the location
information up-to-date, you should normally set followUserLocation
as well.
Working with maps
The previous map displayed a single marker showing the location of your device. It would be handy to add
other features to the map. Built-in to the MapView
component, the user's current location
and points of interest around the user can be rendered. You will probably want to add additional points
of interest that are relevant to your application, instead of the points of interest that are rendered
by default. Let's begin by adding additional points to the map.
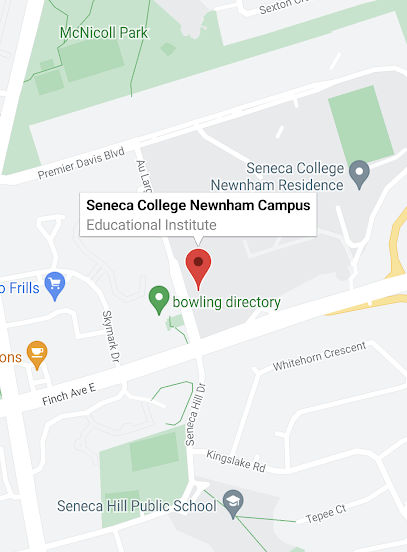
You can also render polygon features as well. Here is an example.
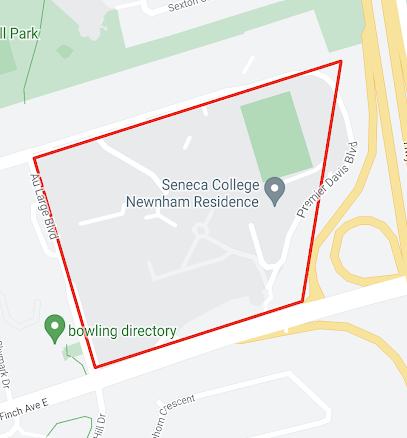
You can learn more about the MapView
component and features by visiting the
react-native-maps GitHub page.