Day 7 - July 6, 2022
Topics Covered
- Working with item lists
- Sorting and filtering lists
- Fetching list data
- Lazy loading of list data
Working with item lists
Previously, we were introduced to the FlatList
component. This component allows
applications to render lists on both iOS and Android. Passing an array to the data
property of the FlatList
is all that is required to get started. The array can contain as
many JavaScript objects as you want rendered. Those objects can contain any properties you want however
they must at minimum contain a property called key
. Failure to include this property will
result in errors during render.
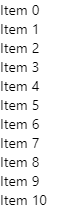
We start off by defining an array of items. To save some coding, fill
is used create ten
objects in the array and map
is used to generate default properties for each object. Notice
each object has a key
property.
Next, we render the FlatList
by passing the data
property the array of
objects. We also set the renderItem
property. This property effectively contains a UI
template to be used in place of each object. We are simply using a <Text>
component
but any component could have been used. In fact, multiple components could be used but remember that you
must have a root element to contain the components.
Sorting and filtering lists
The FlatList
component simply renders content for each item in the data
array.
As you manipulate the data source, you change what is rendered on the screen. Changes are not limited to
adding and removing items. Changing the order will also cause the list to render again. Following is an
example. In the example you can press a button to change the sort order and you can type in text to
filter the list.
Fetching list data
It is not uncommon to require data in your applications. In the next code example, we will access a free
REST API to grab and display a list of university names. We use useEffect()
hook to ensure
the
component is ready before asyncronously calling the fetch API to load the list of universities
into the data
state variable. Since this code example builds upon the previous example, we
are able to filter and sort the results.
Lazy loading of list data
The FlatList
implements a handy property called onEndReached
. Using this
property it is easy to implement infinite lists. Think of a Twitter feed. We could load twenty posts on
the initial render, then when the user reaches the bottom, load an additional twenty posts, and so on.
This is called lazy loading and we are effectively growing the list as the user scrolls near the
end.
To load the new items, you will implement the onEndReached
event handler to append
additional data to the end of the list. To do this, you could generate new items programmatically, or
more often, use the Fetch API to load additional results.